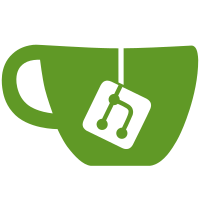
15 changed files with 298 additions and 28 deletions
@ -1,3 +1,5 @@
|
||||
@import "functions/lists"; |
||||
@import "functions/cross_browser_support"; |
||||
@import "functions/gradient_support"; |
||||
@import "functions/constants"; |
||||
@import "functions/display"; |
||||
|
@ -0,0 +1,72 @@
|
||||
// |
||||
// A partial implementation of the Ruby cross browser support functions from Compass: |
||||
// https://github.com/Compass/compass/blob/stable/lib/compass/sass_extensions/functions/cross_browser_support.rb |
||||
// |
||||
|
||||
@function prefixed($prefix, $property1, $property2:null, $property3:null, $property4:null, $property5:null, $property6:null, $property7:null, $property8:null, $property9:null) { |
||||
$properties: $property1, $property2, $property3, $property4, $property5, $property6, $property7, $property8, $property9; |
||||
$prefixed: false; |
||||
@each $item in $properties { |
||||
@if type-of($item) == 'string' { |
||||
$prefixed: $prefixed or str-index($item, 'url') != 1 and str-index($item, 'rgb') != 1 and str-index($item, '#') != 1; |
||||
} @elseif type-of($item) == 'color' { |
||||
} @elseif $item != null { |
||||
$prefixed: true; |
||||
} |
||||
} |
||||
@return $prefixed; |
||||
} |
||||
|
||||
@function prefix($prefix, $property1, $property2:null, $property3:null, $property4:null, $property5:null, $property6:null, $property7:null, $property8:null, $property9:null) { |
||||
$properties: ""; |
||||
|
||||
// Support for polymorphism. |
||||
@if type-of($property1) == 'list' { |
||||
// Passing a single array of properties. |
||||
$properties: $property1; |
||||
} @else { |
||||
// Passing multiple properties. |
||||
$properties: $property1, $property2, $property3, $property4, $property5, $property6, $property7, $property8, $property9; |
||||
} |
||||
|
||||
$props: false; |
||||
@each $item in $properties { |
||||
@if $item == null {} |
||||
@else { |
||||
@if prefixed($prefix, $item) { |
||||
$item: #{$prefix}-#{$item}; |
||||
} |
||||
@if $props { |
||||
$props: $props, $item; |
||||
} |
||||
@else { |
||||
$props: $item; |
||||
} |
||||
} |
||||
} |
||||
@return $props; |
||||
} |
||||
|
||||
@function -svg($property1, $property2:null, $property3:null, $property4:null, $property5:null, $property6:null, $property7:null, $property8:null, $property9:null) { |
||||
@return prefix('-svg', $property1, $property2, $property3, $property4, $property5, $property6, $property7, $property8, $property9); |
||||
} |
||||
|
||||
@function -owg($property1, $property2:null, $property3:null, $property4:null, $property5:null, $property6:null, $property7:null, $property8:null, $property9:null) { |
||||
@return prefix('-owg', $property1, $property2, $property3, $property4, $property5, $property6, $property7, $property8, $property9); |
||||
} |
||||
|
||||
@function -webkit($property1, $property2:null, $property3:null, $property4:null, $property5:null, $property6:null, $property7:null, $property8:null, $property9:null) { |
||||
@return prefix('-webkit', $property1, $property2, $property3, $property4, $property5, $property6, $property7, $property8, $property9); |
||||
} |
||||
|
||||
@function -moz($property1, $property2:null, $property3:null, $property4:null, $property5:null, $property6:null, $property7:null, $property8:null, $property9:null) { |
||||
@return prefix('-moz', $property1, $property2, $property3, $property4, $property5, $property6, $property7, $property8, $property9); |
||||
} |
||||
|
||||
@function -o($property1, $property2:null, $property3:null, $property4:null, $property5:null, $property6:null, $property7:null, $property8:null, $property9:null) { |
||||
@return prefix('-o', $property1, $property2, $property3, $property4, $property5, $property6, $property7, $property8, $property9); |
||||
} |
||||
|
||||
@function -pie($property1, $property2:null, $property3:null, $property4:null, $property5:null, $property6:null, $property7:null, $property8:null, $property9:null) { |
||||
@return prefix('-pie', $property1, $property2, $property3, $property4, $property5, $property6, $property7, $property8, $property9); |
||||
} |
@ -0,0 +1,15 @@
|
||||
/* |
||||
* A partial implementation of the Ruby gradient support functions from Compass: |
||||
* https://github.com/Compass/compass/blob/v0.12.2/lib/compass/sass_extensions/functions/gradient_support.rb |
||||
*/ |
||||
|
||||
@function color-stops($item1, $item2:null, $item3:null, $item4:null, $item5:null, $item6:null, $item7:null, $item8:null, $item9:null) { |
||||
$items: $item2, $item3, $item4, $item5, $item6, $item7, $item8, $item9; |
||||
$full: $item1; |
||||
@each $item in $items { |
||||
@if $item != null { |
||||
$full: $full, $item; |
||||
} |
||||
} |
||||
@return $full; |
||||
} |
@ -0,0 +1,13 @@
|
||||
var render = require('../helper/render'); |
||||
var ruleset = require('../helper/ruleset'); |
||||
|
||||
describe("CSS3 Border Radius", function () { |
||||
|
||||
it("should generate a border radius", function (done) { |
||||
render(ruleset('$experimental-support-for-mozilla: false; $experimental-support-for-opera: false; @include border-radius(0, 0);'), function(output, err) { |
||||
expect(output).toBe(ruleset('-webkit-border-radius:0 0;border-radius:0 / 0;')); |
||||
done(); |
||||
}, ['compass/css3/border-radius']); |
||||
}); |
||||
|
||||
}); |
@ -0,0 +1,13 @@
|
||||
var render = require('../helper/render'); |
||||
var ruleset = require('../helper/ruleset'); |
||||
|
||||
describe("CSS3 Box Shadow", function () { |
||||
|
||||
it("should generate a default box shadow", function (done) { |
||||
render(ruleset('$default-box-shadow-inset: inset; $default-box-shadow-h-offset: 23px; $default-box-shadow-v-offset: 24px; $default-box-shadow-blur: 17px; $default-box-shadow-spread: 15px; $default-box-shadow-color: #DEADBE; $experimental-support-for-mozilla: false; $experimental-support-for-opera: false; @include box-shadow;'), function(output, err) { |
||||
expect(output).toBe(ruleset('-webkit-box-shadow:inset 23px 24px 17px 15px #DEADBE;box-shadow:inset 23px 24px 17px 15px #DEADBE;')); |
||||
done(); |
||||
}, ['compass/css3/box-shadow']); |
||||
}); |
||||
|
||||
}); |
@ -0,0 +1,27 @@
|
||||
var render = require('../helper/render'); |
||||
var ruleset = require('../helper/ruleset'); |
||||
|
||||
describe("CSS3 Images", function () { |
||||
|
||||
it("should generate a background", function (done) { |
||||
render(ruleset('@include background(ok);'), function(output, err) { |
||||
expect(output).toBe(ruleset('background:-owg-ok;background:-webkit-ok;background:-moz-ok;background:-o-ok;background:ok;')); |
||||
done(); |
||||
}, ['compass/css3/images']); |
||||
}); |
||||
|
||||
it("should generate multiple backgrounds", function (done) { |
||||
render(ruleset('$support-for-original-webkit-gradients: false; $experimental-support-for-mozilla: false; $experimental-support-for-opera: false; @include background(a, b, c);'), function(output, err) { |
||||
expect(output).toBe(ruleset('background:-webkit-a,-webkit-b,-webkit-c;background:a,b,c;')); |
||||
done(); |
||||
}, ['compass/css3/images']); |
||||
}); |
||||
|
||||
it("should generate multiple backgrounds of different types", function (done) { |
||||
render(ruleset('$support-for-original-webkit-gradients: false; $experimental-support-for-mozilla: false; $experimental-support-for-opera: false; @include background(#fff, url(1.gif), linear-gradient(white, black));'), function(output, err) { |
||||
expect(output).toBe(ruleset('background:#fff,url(1.gif),-webkit-linear-gradient(#ffffff, #000000);background:#fff,url(1.gif),linear-gradient(#ffffff, #000000);')); |
||||
done(); |
||||
}, ['compass/css3/images']); |
||||
}); |
||||
|
||||
}); |
@ -0,0 +1,13 @@
|
||||
var render = require('../helper/render'); |
||||
var ruleset = require('../helper/ruleset'); |
||||
|
||||
describe("CSS3 Transition", function () { |
||||
|
||||
it("should generate a transition", function (done) { |
||||
render(ruleset('$experimental-support-for-mozilla: false; $experimental-support-for-opera: false; @include transition(ok 0s);'), function(output, err) { |
||||
expect(output).toBe(ruleset('-webkit-transition:ok 0s;transition:ok 0s;')); |
||||
done(); |
||||
}, ['compass/css3/transition']); |
||||
}); |
||||
|
||||
}); |
@ -0,0 +1,3 @@
|
||||
module.exports = function(prop) { |
||||
return 'a{b:'+prop+';}'; |
||||
} |
@ -0,0 +1,19 @@
|
||||
var sass = require('node-sass'); |
||||
var libDir = __dirname.replace(/test\/helper$/, 'lib'); |
||||
var chalk = require('chalk'); |
||||
|
||||
module.exports = function(data, callback, imports) { |
||||
imports = imports ? imports.map(function(i){ return '@import "'+libDir+'/'+i+'";'}) : []; |
||||
|
||||
sass.render({ |
||||
data: '@import "'+libDir+'/compass/functions";' + imports.join('') + data, |
||||
outputStyle: 'compressed', |
||||
success: function(output){ |
||||
callback(output); |
||||
}, |
||||
error: function(err){ |
||||
console.log(chalk.red("Sass error:"), err); |
||||
callback('', err); |
||||
} |
||||
}); |
||||
} |
Loading…
Reference in new issue