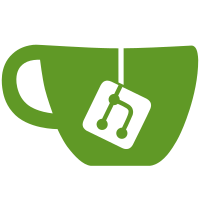
6 changed files with 101 additions and 35 deletions
@ -0,0 +1,20 @@
|
||||
var render = require('../helper/render'); |
||||
var ruleset = require('../helper/ruleset'); |
||||
|
||||
describe("CSS3 Images", function () { |
||||
|
||||
it("should generate a background", function (done) { |
||||
render(ruleset('@include background(ok);'), function(output, err) { |
||||
expect(output).toBe(ruleset('background:-owg-ok;background:-webkit-ok;background:-moz-ok;background:-o-ok;background:ok;')); |
||||
done(); |
||||
}, ['compass/css3/images']); |
||||
}); |
||||
|
||||
it("should generate multiple backgrounds", function (done) { |
||||
render(ruleset('$support-for-original-webkit-gradients: false; $experimental-support-for-mozilla: false; $experimental-support-for-opera: false; @include background(a, b, c);'), function(output, err) { |
||||
expect(output).toBe(ruleset('background:-webkit-a,-webkit-b,-webkit-c;background:a,b,c;')); |
||||
done(); |
||||
}, ['compass/css3/images']); |
||||
}); |
||||
|
||||
}); |
@ -0,0 +1,3 @@
|
||||
module.exports = function(prop) { |
||||
return 'a{b:'+prop+';}'; |
||||
} |
@ -0,0 +1,19 @@
|
||||
var sass = require('node-sass'); |
||||
var libDir = __dirname.replace(/test\/helper$/, 'lib'); |
||||
var chalk = require('chalk'); |
||||
|
||||
module.exports = function(data, callback, imports) { |
||||
imports = imports ? imports.map(function(i){ return '@import "'+libDir+'/'+i+'";'}) : []; |
||||
|
||||
sass.render({ |
||||
data: '@import "'+libDir+'/compass/functions";' + imports.join('') + data, |
||||
outputStyle: 'compressed', |
||||
success: function(output){ |
||||
callback(output); |
||||
}, |
||||
error: function(err){ |
||||
console.log(chalk.red("Sass error:"), err); |
||||
callback('', err); |
||||
} |
||||
}); |
||||
} |
Loading…
Reference in new issue